๋ฐ๋ผํ๋ฉด ๋๋ Netty ๊ธฐ๋ฐ ๊ฐ๋จํ Live Chat ์๋ฒ ์์
๐ก ๋น๋๊ธฐ ์ด๋ฒคํธ ๊ธฐ๋ฐ ๋คํธ์ํฌ ์ ํ๋ฆฌ์ผ์ด์
ํ๋ ์์ํฌ์ธ Netty๋ฅผ ํ์ตํ๋ฉฐ Simple Chat Server๋ฅผ ๊ตฌ์ถํ ์ ์๋ค.
์ง๋๋ฒ ๋ง๋ค์๋ EchoServer๋ฅผ ์ฌํํด Live Chat ์๋ฒ๋ฅผ ๊ตฌํํด๋ณด์
์ ๋ฒ ์์ ๋ EchoServer.java์ EchoServerHandler.java ํ์ผ๋ก ๊ตฌ์ฑ๋์์ง๋ง,
์ด๋ฒ ์์ ์ ํ์ผ ๊ตฌ์ฑ์ ์๋์ ๊ฐ๋ค.
- ChatServer.java
- ChatServerHandler.java
- ChatClient.java
- ChatClientHandler.java
ChatServer.java
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelPipeline;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioServerSocketChannel;
import io.netty.handler.codec.string.StringDecoder;
import io.netty.handler.codec.string.StringEncoder;
public final class ChatServer {
static final int PORT = 8007;
public static void main(String[] args) throws Exception {
EventLoopGroup bossGroup = new NioEventLoopGroup(1);
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup) // Set boss & worker groups
.channel(NioServerSocketChannel.class)// Use NIO to accept new connections.
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline p = ch.pipeline();
p.addLast(new StringDecoder());
p.addLast(new StringEncoder());
// This is our custom server handler which will have logic for chat.
p.addLast(new ChatServerHandler());
}
});
// Start the server.
ChannelFuture f = b.bind(PORT).sync();
System.out.println("Chat Server started. Ready to accept chat clients.");
// Wait until the server socket is closed.
f.channel().closeFuture().sync();
} finally {
// Shut down all event loops to terminate all threads.
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
}
ChatServerHandler.java
import java.util.ArrayList;
import java.util.List;
import io.netty.channel.Channel;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.SimpleChannelInboundHandler;
public class ChatServerHandler extends SimpleChannelInboundHandler<String> {
static final List<Channel> channels = new ArrayList<Channel>();
@Override
public void channelActive(final ChannelHandlerContext ctx) {
System.out.println("Client joined - " + ctx);
channels.add(ctx.channel());
}
@Override
public void channelRead0(ChannelHandlerContext ctx, String msg) throws Exception {
System.out.println("Server received - " + msg);
for (Channel c : channels) {
c.writeAndFlush("-> " + msg + '\n');
}
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) {
System.out.println("Closing connection for client - " + ctx);
ctx.close();
}
}
ChatClient.java
import java.util.Scanner;
import io.netty.bootstrap.Bootstrap;
import io.netty.channel.Channel;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelPipeline;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioSocketChannel;
import io.netty.handler.codec.string.StringDecoder;
import io.netty.handler.codec.string.StringEncoder;
public class ChatClient {
static final String HOST = "127.0.0.1";
static final int PORT = 8007;
static String clientName;
public static void main(String[] args) throws Exception {
Scanner scanner = new Scanner(System.in);
System.out.println("Please enter your name: ");
if (scanner.hasNext()) {
clientName = scanner.nextLine();
System.out.println("Welcome " + clientName);
}
EventLoopGroup group = new NioEventLoopGroup();
try {
Bootstrap b = new Bootstrap();
b.group(group) // Set EventLoopGroup to handle all eventsf for client.
.channel(NioSocketChannel.class)// Use NIO to accept new connections.
.handler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline p = ch.pipeline();
p.addLast(new StringDecoder());
p.addLast(new StringEncoder());
// This is our custom client handler which will have logic for chat.
p.addLast(new ChatClientHandler());
}
});
// Start the client.
ChannelFuture f = b.connect(HOST, PORT).sync();
while (scanner.hasNext()) {
String input = scanner.nextLine();
Channel channel = f.sync().channel();
channel.writeAndFlush("[" + clientName + "]: " + input);
channel.flush();
}
// Wait until the connection is closed.
f.channel().closeFuture().sync();
} finally {
// Shut down the event loop to terminate all threads.
group.shutdownGracefully();
}
}
}
ChatClientHandler.java
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.SimpleChannelInboundHandler;
public class ChatClientHandler extends SimpleChannelInboundHandler<String> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, String msg) throws Exception {
System.out.println("Message: " + msg);
}
}
1. ChatServer.java๋ฅผ Runํ๋ค.

2. ChatClient.java๋ฅผ Runํ๋ค.
3. ์ด๋ฆ์ ์ ๋ ฅํ๋ค.
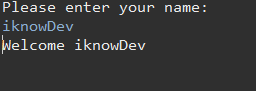

4. iknowDev๊ฐ hello world๋ผ๊ณ ์ ๋ ฅํ๋ค.
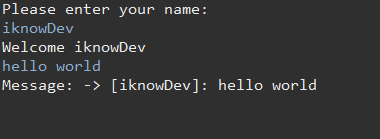

5. ClientServer๋ฅผ ํ๋ ๋ Runํ๊ณ ์ฌ๋ฌ ์ฌ์ฉ์์ ๋น๋๊ธฐ ๋ํ๋ฅผ ํ์ธํ ์ ์๋ค.
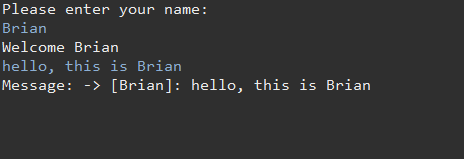


Netty Project | Understanding Netty using simple real-world example of Chat server client | Good for beginners - Its All Binary
In this article we will understand Netty in simplistic way with real world example of Chat server client example. What…
itsallbinary.com